OpenUSD Library Tour
OpenUSD has a lot of libraries, each with a different weird and mysterious name. Let's take a tour!
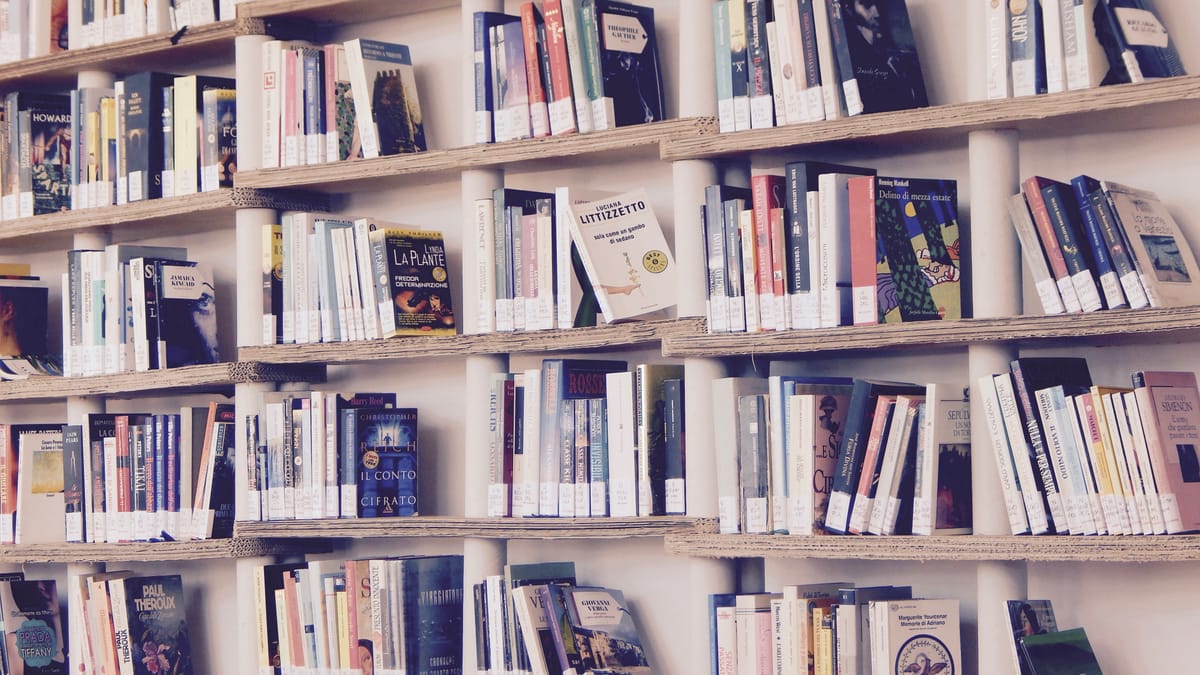
OpenUSD has a lot of libraries, each with a different weird and mysterious name. Let's take a tour!
The Landscape
Long ago when I worked at Pixar we had three regions of the codebase, called bedrock, amber and lava.
Bedrock was stable code that everything else depended on, so it changed rarely and when it did there was upheaval. Bedrock could only use code from bedrock.
Amber was where most software engineers worked, it was less stable than bedrock so you could move faster (relatively). It could use code from amber or bedrock.
Lava was the least stable and was where artists wrote code and scripts. It could use code from anywhere, and when it worked it was an exciting frontier where some of the best stuff was prototyped.
USD's top level source folders are organized with a similar philosophy. In the pxr folder you have
- base This is like bedrock. It's the foundation, and things in base can only depend on other things in base. There is very little graphics here, it's mostly fundamental math, multiplatform support, stuff like that.
- usd This is where the core usd functionality lives. The usd file types, livrps algorithm, asset resolver, graphics prim types, all that's in here. It can only use code from usd and base.
- imaging This is the core of Hydra, and has all the fundamental rendering code. Can depend on imaging, base or usd.
- usdImaging The highest level code, can depend on everything else. This has Hydra delegates, usdview, etc.
Let's go through these folders in order and take a really quick look at what each one provides.
The Dungeon
We'll start our tour in base, which I just decided to call the dungeon. These are the shifty miscreants who are imprisoned here.
⭐ arch 🔹 Architecture
Platform specific code, and a few very low level utilities for things like malloc hooks.
⭐ gf 🔹 Graphics Foundation
The main math library. Provides types for linear algebra and for specific graphics needs like cameras and frustums.
⭐ js 🔹 JSON
JSON file reader/writer, built on rapidjson. This is only used in C++, python should use the python json library.
⭐ plug 🔹 Plugin
The plugin system. Loads plugins and handles reading the pluginfo.json files USD uses.
⭐ tf 🔹 Tools Foundation
A large set of reusable C++ classes and code.
Tf provides the basic features for providing python bindings and for hosting python interpreters.
It has a logging system, a runtime type system, string formatting, the token type for registering strings, a reference counted pointer type, a notification callback system, and utilities for mutexes, RAII objects, environment variables, file i/o... I'm sure I missed a few things.
⭐ trace 🔹 Performance Tracing
A system for tracking performance using instrumented functions. USD has a lot of this instrumentation built-in, so enabling this can give good performance feedback. Trace can output traces in chrome tracing format.
⭐ ts 🔹 🚧 Animation Splines
This is extremely new as of the time of writing and is still in flux. Ts provides support for a spline value object, which USD hasn't supported until now. Previously you would need to roll your own solution, or use timesampled animation instead of splines.
I honestly have no idea what the T stands for.
⭐ vt 🔹 Value Types
Provides dynamically sized containers for plain old data types and Gf math types. Vt arrays and other sized types are copy-on-write, saving a lot of time passing buffers around, and can even support copy free passing of buffers to and from python.
Vt also provides a type-erased value type VtValue
that is used often in USD apis, especially for communicating with Python. VtDictionary
and VtValue
together can marshal Python dictionaries to and from C++
⭐ work 🔹 Work (Multithreading)
Wraps TBB to offer some easily called multithreading utilities. Also manages dispatch and the thread pool
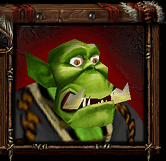
The Castle
Let's stick with this medieval architecture metaphor, I'm having fun. Who lives on the ground floor, lording it over the dungeon? These hard working yeomen! In usd there is
⭐ ar 🔹 Asset Resolver
Implements the asset resolver base classes users can derive from, and provides a default resolver. Resolvers take paths from usd composition arcs and remap them to the layers you actually open.
⭐ bin 🔹 Binary (Utilities)
Has some python apps and some that require C++. usdcat
, usdedit
and other applications that don't use imaging are all here.
⭐ kind 🔹 Kind
Kind is a sort of type system for telling which prims are models, groups of models, etc. The available kinds are extensible.
Most terms for type systems are already taken. If you've already got class, model, type, what can you call this? This is called "kind" in the sense of "what kind of thing is it?" "Oh, it's that kind."
⭐ ndr 🔹 Node Definition Registry
An extremely generic library for storing node types, of the sort you might use in a shading graph, or composition, etc. Underpins several other libraries that need to have nodes with properties and connections.
⭐ pcp 🔹 Prim Cache Population
This is a cache for what USD calls "composition." When you load a USD Stage and it reads in a network of layers for your scene, pcp caches the traversal information and data so that lookups are fast.
⭐ plugin 🔹 File Type Plugins
Contains some of the file type plugins USD ships with. Has support for Alembic, Osl and Draco files at the time of writing.
⭐ sdf 🔹 Scene Description Foundation
Usd is the library that deals with whole stages and scenes, which are collections of files. Sdf is the library that deals with individual files only. If you use Sdf to open a Usd file that has references the references are not read in. You open only that file, not a stage.
Sdf also provides the SdfPath type, defines what value types are valid in Usd files, and defines the text usda
format.
⭐ sdr 🔹 Shader Definition Registry
Builds on ndr to create nodes that are suitable for shader networks. Sdr is shading language agnostic, it just stores node networks without understanding the shaders that underpin them. It's up to the imaging code to turn these into real shaders.
⭐ usd 🔹 Universal Scene Description
Usd has the Stage type, and defines the basic Usd Prim type, Usd Property and Attribute types, utilities for stage traversal and editing, and much more. The Usd library is the core feature-set of OpenUsd as a whole.
⭐ usdXXX 🔹 Quite a few libs providing prim types
I'm not going to break these out individually, because there are lots and this is already too long. Each of these provides concrete schemas for USD prim types. Some highlights
- usdGeom 🔸 Mesh, Xform, Sphere, ...
- usdShade 🔸 Material, Shader, ...
- usdLux 🔸 DistantLight, DomeLight, ...
- usdSkel 🔸 Skeleton, BlendShape
The Ramparts
The next layer up is imaging. This is the core of Hydra, the imaging system that ships with USD. There's a lot of these guys defending the walls, so I'm going to bundle a few related ones, I recommend skimming and only reading the interesting ones. There's
⭐ cameraUtil 🔹 Camera Utilities
GfCamera provides low level math for cameras, this library provides utilities for modeling more real world cameras. There's math related to filmback, output windows, and other practical things.
⭐ glarch 🔹 OpenGL Architecture
Platform wrappers for OpenGL. This is used in the Storm Hydra render delegate, which renders using OpenGL.
⭐ geomUtil 🔹 Geometry Utilities
Utilities for generating things like cones, cuboids, cylinders. Note that this does not generate USD prims, only geometry for rendering.
⭐ glf 🔹 OpenGL Foundation
Code for managing glContexts, draw targets, materials, etc. The main OpenGL driving classes for Storm.
⭐ hd 🔹 Hydra
This is the core hydra library. Hydra is a framework that takes one or more scene graphs as input and converts them into data fed to one or more renderer. It's used as a plugin in many digital content creation tools to display USD viewport renders.
⭐ hdGp 🔹 Hydra Generative Procedural
A Hydra 2.0 feature. Generate procedural information at render time.
⭐ hdMtlx 🔹 Hydra MaterialX
Utilities for working with MaterialX shader networks.
⭐ hdSt 🔹 Hydra Storm
The core OpenGL renderer used by the Hydra Storm render delegate. Note that the delegate plugin itself is in the imaging/plugin/hdStorm folder.
⭐ hdar 🔹 Hydra Asset Resolver
A Hydra 2.0 utility to assist with Usd asset resolution.
⭐ hdsi 🔹 Hydra Scene Indices
A Hydra 2.0 library that provides a variety of scene index types.
⭐ hdx 🔹 Hydra Extensions
Useful utilities for applications that embed Hydra. Code for fullscreen shaders, selection in a viewport, colorizing, etc.
⭐ hf 🔹 Have Fun? (it's a plugin system)
Provides a plugin system for Hydra, using the base/plug library underneath.
I honestly don't know what this one stands for, and perusing the source didn't help.
⭐ hgi🔹 Hydra Graphics Interface
Base classes for wrapping a GPU library like OpenGL, Vulkan, Metal, etc. Provides a common api for Hydra to use.
⭐hgiGL, hgiMetal, hgiVulkan, etc
Hgi implementations for these graphics APIs.
⭐ hio 🔹 Hydra Input/Output (and hioOpenVDB)
Code for reading glslfx files, OpenVDB files, images (using openimageio or stb)
⭐ plugin 🔹 Hydra Plugins
Render delegates for Storm, Embree and Prman are in here
⭐ pxOsd 🔹 Pixar OpenSubdiv
Support for working with OpenSubdiv for subdivision surfaces
The Tower
Finally we get to the top, with the flashy wizards in the tower. Yeah, their magic is cool, but could they do it without the rest of the castle? I think not.
usdImaging contains
⭐ bin 🔹 Binary (Utilities)
usdview, usdrecord, and other helpful applications.
⭐ plugin 🔹 Plugins
Contains high level shader plugins. The reference implementation for UsdPreviewShader is here, along with some other interesting code.
⭐ usdAppUtils
These are helpers used in usdview and usdrecord
⭐ usdImaging 🔹A Scene Delegate
This is the Hydra 1.0 Scene Delegate that ships with USD. It's what feeds USD stages into Hydra for rendering, and it's used in usdview and many other real time USD renderers. It still works with Hydra 2.0, but in the future may be replaced by a higher performance alternative built on Hydra 2.0 natively.
⭐ usdImagingGL 🔹 A Rendering Engine
This is the rendering engine usdview uses. It manages a Hydra instance and does things like highlight selection, implement picking and switch out the render delegates when requested.
I find the naming of this library a little strange. It isn't a version of the usdImaging scene delegate, but has a very similar name. It also isn't limited to OpenGL rendering. I suspect its just a legacy name. In any case, it's pretty cool.
⭐ usdProcImaging 🔹 USD Procedural Imaging
This is a Hydra plugin that implements a "display adapter" for procedurals. Display adapters are plugins to Hydra that tell it how to render a type of Prim.
⭐ usdRiPxrImaging 🔹 USD Renderman Pixar Imaging
Hydra display adapters and filters for rendering with Renderman.
⭐usdSkelImaging 🔹 USD Skeleton Imaging
Hydra display adapters for showing skeleton guides
⭐usdVolImaging 🔹 USD Volume Imaging
Hydra display adapters for rendering Volumes. Field3d and OpenVDB volumes are supported.
⭐usdviewq 🔹 UsdView Qt
The Qt part of usdview. The UI and interactive parts of usdview are implemented here. The actual script that launches usdview is in usdImaging/bin
Please Exit Through The Gift Shop
This concludes our tour of castle pxr. I hope it was interesting, and sincerely hope you didn't read the whole thing 😆